How to Install and Use ConcurrentQueue for Enhanced Performance π
Saturday, Dec 21, 2024 | 8 minute read
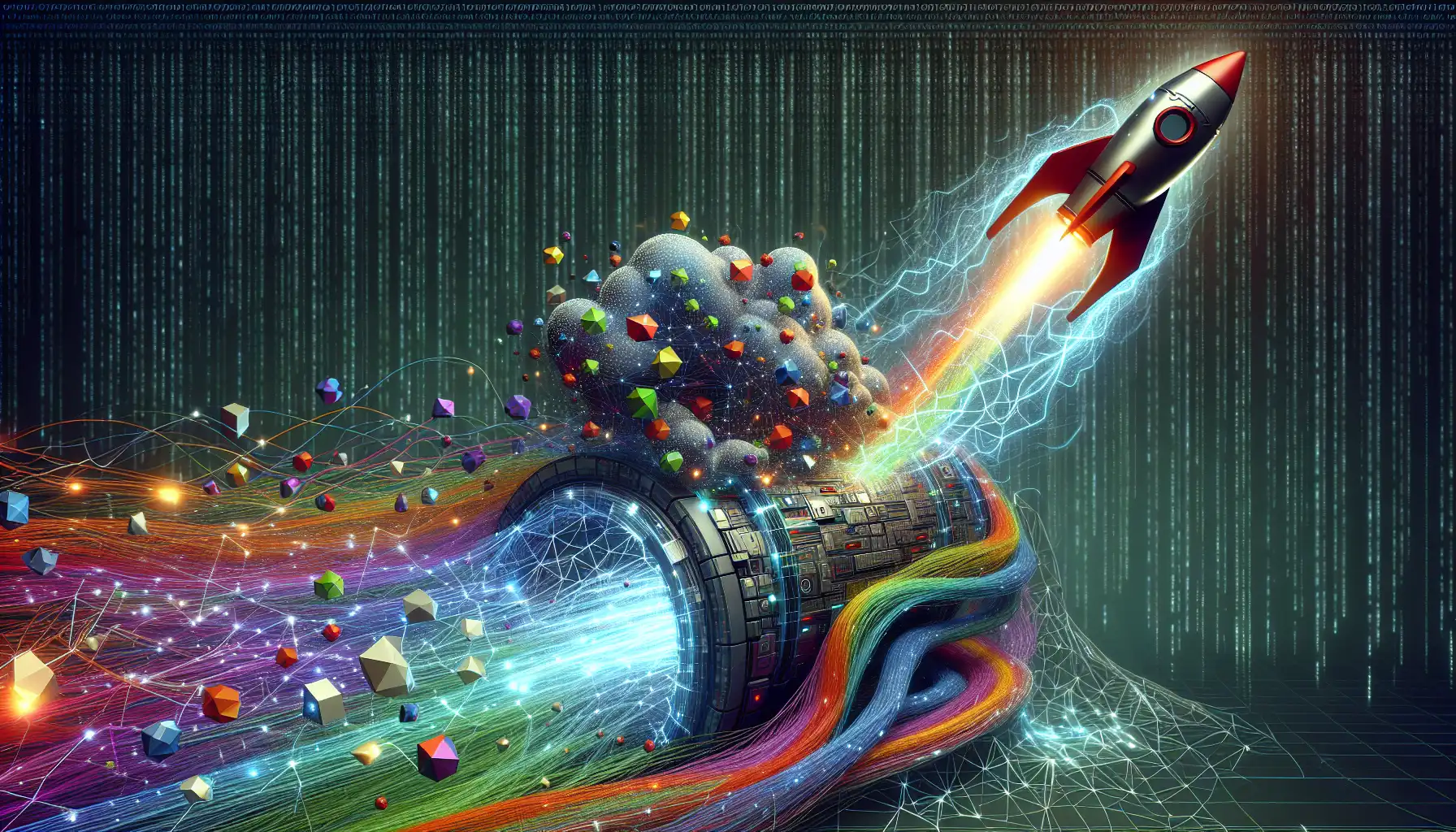
Unlock Lightning-Fast Concurrency! β‘ Experience a high-performance, lock-free queue that enhances efficiency in multi-threaded applications, ensures thread safety, and supports unlimited item types. Perfect for developers aiming to tackle concurrency with ease! π
“In this fast-paced digital age, effectively handling concurrency issues has become a new challenge for software developers.” π
In modern software development, particularly in high-performance real-time applications, managing data exchange in a multi-threaded environment efficiently is crucial. To tackle this challenge, developers need a tool that can handle multi-threaded operations simultaneously and improve data processing efficiency. Enter ConcurrentQueueβan exceptional open-source library that excels in this realm, opening a new era of efficient concurrency handling with its lock-free characteristics and outstanding performance! π
1. What is ConcurrentQueue? π A High-Performance Lock-Free Concurrent Queue
moodycamel::ConcurrentQueue is a high-performance lock-free concurrent queue designed for C++11. It enables multiple threads to operate simultaneously as both producers and consumers, significantly enhancing efficiency. Thanks to its single-header design, users can easily integrate it into existing projects and swiftly achieve efficient data processing. Whether in game development, financial computing, or network servers, ConcurrentQueue can deliver its unique value. πͺ
2. The Unique Charm of ConcurrentQueueβKey Features Explained β¨
-
Performance: ConcurrentQueue optimizes enqueue and dequeue operations to ensure ultra-fast processing speeds, allowing you to handle high-concurrency scenarios with ease. β‘
-
Thread Safety: This queue provides complete thread safety, allowing any number of threads to use it without worrying about data races, thus boosting the efficiency of concurrent processing. π
-
Memory Management: Its template-based design supports static and dynamic memory allocation, greatly reducing dependency on pointers, making memory management more flexible and secure. π§©
-
Unlimited: The design of ConcurrentQueue does not impose limits on element types or the maximum number of items in the queue. Users can expand as needed to fully meet various application scenarios. π
-
Batch Operations: It offers efficient bulk enqueue and dequeue functionalities, maintaining high performance even in competitive situations, making it an essential tool for writing efficient code. π
-
Blocking Version: If you need consumers to wait for new items to arrive, the BlockingConcurrentQueue variation is perfect for that requirement. π₯
-
Exception Safety: When handling types that may throw exceptions, ConcurrentQueue still maintains stable performance, effectively avoiding data corruption and enhancing the robustness of the entire system. π§
3. Developer’s Preferred ChoiceβWhy Choose ConcurrentQueue? β€οΈ
Developers favor moodycamel::ConcurrentQueue primarily for its combination of lock-free concurrency and high performance, along with a flexible memory management solution. Whether in complex concurrent programming environments or in the face of rapid data processing requirements, ConcurrentQueue provides satisfactory solutions. It is the ideal choice for multi-producer, multi-consumer concurrent queues, allowing developers to engage in multi-threaded programming more efficiently and often achieve their goals with ease. π
In summary, ConcurrentQueue is not just a simple tool; it is a secret weapon for enhancing software performance! Experience this incredibly attractive concurrent queue and drive your projects toward greater efficiency and performance! π
π Installing ConcurrentQueue π οΈ
Installing ConcurrentQueue is super simple! You can easily get it done in a few ways:
1. Using vcpkg π
Open your terminal and execute the following commands:
git clone https://github.com/Microsoft/vcpkg.git
cd vcpkg
./bootstrap-vcpkg.sh
./vcpkg integrate install
vcpkg install concurrentqueue
Here’s a little breakdown of these commands:
git clone
clones the vcpkg repository to your local machine, enabling easy management of C++ library installations.cd vcpkg
switches to the vcpkg directory../bootstrap-vcpkg.sh
runs this script to download and compile the necessary files, ensuring that you can use this tool../vcpkg integrate install
integrates vcpkg with your development environment to ensure it can automatically find the installed libraries.vcpkg install concurrentqueue
is the crucial step to actually install the ConcurrentQueue library.
2. Using FreeBSD Ports π¦
You can also install it using ports on FreeBSD systems:
cd /usr/ports/devel/concurrentqueue/ && make install clean
Hereβs what this does:
cd /usr/ports/devel/concurrentqueue/
switches to the ports directory for ConcurrentQueue.make install clean
compiles and installs the library files based on the Makefile configurations, then cleans up temporary files.
3. Using pkg π
On FreeBSD, you can quickly install it via pkg:
pkg install devel/concurrentqueue
pkg install concurrentqueue
Using pkg install
, simply specify the library you want to install, and pkg will handle all dependencies and complete the installation in one go!
With any of these methods, you can swiftly start using ConcurrentQueue and experience its powerful concurrency handling capabilities!
π Basic Usage
Letβs take a look at a basic example of using the concurrent queue! β¨
#include "concurrentqueue.h" // Import the concurrent queue header file
moodycamel::ConcurrentQueue<int> q; // Declare a concurrent queue for integers
q.enqueue(25); // Enqueue the number 25
int item; // Declare an integer variable to store dequeued elements
bool found = q.try_dequeue(item); // Attempt to dequeue an element from the queue
assert(found && item == 25); // Ensure the dequeued element is 25
In this example, we:
- Use the
#include
statement to import the ConcurrentQueue header file to access its powerful features. - Create a concurrent queue object
q
for integers so that you can enjoy multi-threaded queue operation support. - Use the
enqueue
method to enqueue the number25
. - Declare an integer variable
item
to receive the dequeued element. - Attempt to dequeue using the
try_dequeue
method, where the returned valuefound
indicates whether the dequeue operation was successful, and we useassert
to verify the correctness of the dequeued result.
βοΈ Through this simple example, you can quickly grasp the basic operations of ConcurrentQueue and easily implement data enqueuing and dequeuing!
π½οΈ Producer-Consumer Pattern
Next, letβs implement a classic producer-consumer model with ConcurrentQueue:
#include "blockingconcurrentqueue.h" // Import the blocking concurrent queue header file
moodycamel::BlockingConcurrentQueue<int> q; // Declare a blocking concurrent queue
// Create a producer thread
std::thread producer([&]() {
for (int i = 0; i != 100; ++i) {
std::this_thread::sleep_for(std::chrono::milliseconds(i % 10)); // Simulate work delay
q.enqueue(i); // Enqueue data
}
});
// Create a consumer thread
std::thread consumer([&]() {
for (int i = 0; i != 100; ++i) {
int item; // Store the dequeued element
q.wait_dequeue(item); // Block until there is an element to dequeue
assert(item == i); // Verify the dequeued element matches the expected value
// Try dequeue with a timeout
if (q.wait_dequeue_timed(item, std::chrono::milliseconds(5))) {
++i; // Increment if dequeue was successful
assert(item == i); // Verify the dequeued element matches the expected value
}
}
});
producer.join(); // Wait for the producer thread to finish
consumer.join(); // Wait for the consumer thread to finish
assert(q.size_approx() == 0); // Verify that the queue is empty
In this example, we created a producer thread and a consumer thread:
- The producer thread simulates processing delays using
std::this_thread::sleep_for
and enqueues numbers from0
to99
. - The consumer thread blocks waiting for available elements in the queue with
wait_dequeue
. - After every dequeue, the consumer verifies the dequeued element against the loop variable
i
usingassert
. - The
wait_dequeue_timed
method attempts to dequeue with a timeout, allowing the consumer to verify after expiration if the queue is exhausted.
π§ This pattern is an effective classic design method in multi-threaded programming that enables efficient utilization of shared queues for task production and consumption.
π Using Tokens for Producer Patterns
ConcurrentQueue also supports using tokens for finer-grained control over enqueuing and dequeuing:
moodycamel::ConcurrentQueue<int> q; // Declare a concurrent queue for integers
moodycamel::ProducerToken ptok(q); // Create a producer token
q.enqueue(ptok, 17); // Enqueue the number 17 using the token
moodycamel::ConsumerToken ctok(q); // Create a consumer token
int item;
q.try_dequeue(ctok, item); // Attempt to dequeue using the token
assert(item == 17); // Verify that the dequeued element is 17
The key points here:
- ProducerToken enables safe enqueuing of data in a specific context.
- Using
enqueue(ptok, 17)
ensures thread safety. - ConsumerToken allows dequeuing within a specific context, effectively ensuring safety in multi-threaded operations.
- The method
try_dequeue(ctok, item)
attempts to dequeue and confirms the accuracy of the result.
π This mechanism enhances concurrent safety, ensuring that operations from multiple threads do not interfere with each other.
πββοΈ Batch Enqueuing and Dequeuing
ConcurrentQueue also supports batch operations, enabling you to handle multiple elements more efficiently:
moodycamel::ConcurrentQueue<int> q; // Declare a concurrent queue for integers
int items[] = { 1, 2, 3, 4, 5 }; // Define an integer array
q.enqueue_bulk(items, 5); // Batch enqueue multiple elements from the array
int results[5]; // Store results of batch dequeue
size_t count = q.try_dequeue_bulk(results, 5); // Attempt to batch dequeue
for (size_t i = 0; i != count; ++i) {
assert(results[i] == items[i]); // Verify that dequeued elements match the enqueued elements
}
In this example:
- We define an integer array
items
with multiple elements to enqueue. - The
enqueue_bulk(items, 5)
method enqueues the entire array at once, significantly improving operational efficiency. - The
try_dequeue_bulk(results, 5)
method attempts to dequeue elements in bulk, allowing retrieval of multiple items simultaneously. - Finally, we loop through to verify the dequeued results, ensuring each element matches the enqueued data.
π Batch operations can effectively reduce lock contention, making them significantly advantageous when dealing with large data volumes.
π Keep Exploring
With this article’s introduction and examples, you should now be able to quickly get started with ConcurrentQueue! Its design aims to provide efficient multi-threaded queue operations, suitable for producer-consumer models, token usage patterns, and batch enqueuing and dequeuing in various scenarios. We hope you enjoy your experience while using it! π