How to Install and Use spdlog: A High-Speed and Efficient C++ Logging Solution π
Saturday, Jan 11, 2025 | 8 minute read
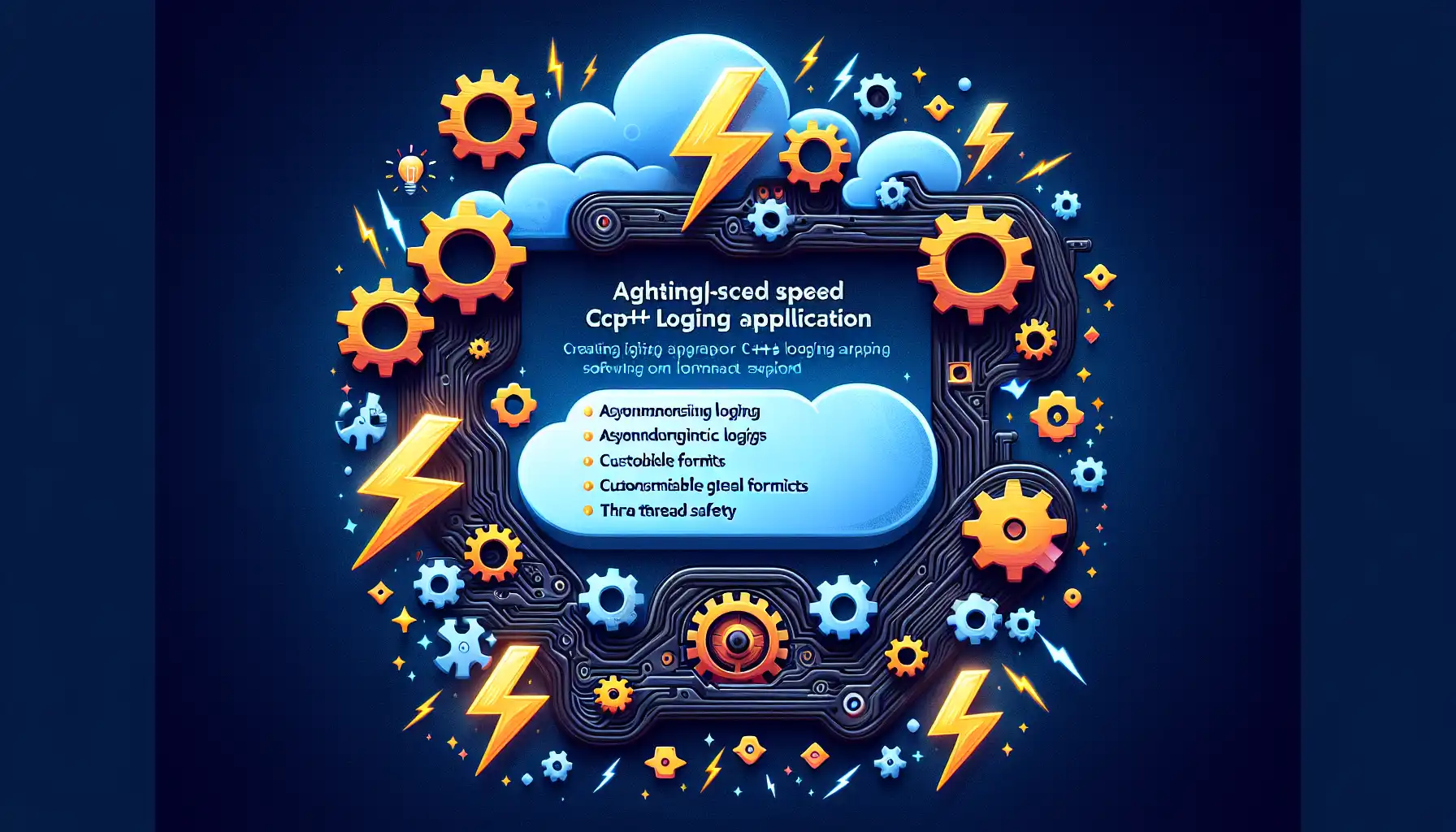
Experience lightning-fast, flexible logging for C++ applications! π Enjoy features like asynchronous logging, customizable formats, thread safety, and a lightweight design for easy integration. Perfect for developers aiming for efficiency and performance! β‘β¨
In this data-driven era, logging systems have become a core component of every application. Efficient logging and management can help developers track errors, optimize performance, and even provide data support for decision-making. As a famous programmer once said: “Logs are not only a written record of issues but also an essential tool in the continual process of optimization and improvement.”
Among numerous C++ logging libraries, spdlog stands out for its exceptional performance and flexible usage. Itβs not only a popular choice among developers but also the perfect solution for efficient log management. Combining high performance, ease of use, and powerful features, spdlog is redefining our expectations of logging systems. β¨
1. Understanding spdlog: The Core of Speed and Efficiency π
- spdlog is an efficient and fast C++ logging library designed for high performance and usability, catering to developersβ high demands for logging systems. With lightning speed and remarkable performance, it shines among many logging libraries.
- The library features a lightweight design, offering both header-only and compiled versions, making it easy for users to integrate into different projects and saving developers valuable time and effort. Whether you’re a beginner or a seasoned developer, spdlog makes it easy to get started!
2. What Sets spdlog Apart: Key Features Unveiled β¨
- High Performance: spdlog’s optimized internal structure makes it a speed leader among various logging libraries. It incurs almost no performance loss while handling logs, keeping your application efficient! π
- Flexible Usage: Users can choose between synchronous or asynchronous logging to adapt to different scenarios. Enjoy flexibility without being confined to a fixed method! π¨
- Diverse Logging Options: It supports logging to rotating files, daily files, console (with color support!), and syslog, empowering you to manage logs in a more diversified and efficient manner! π
- Custom Formatting Ability: Users can customize the output format of logs, offering rich formatting options to meet various application needs, allowing each log to convey more information! π
- Thread Safety: spdlog is optimized for multi-threaded environments, enabling safe logging in both multi-threaded and single-threaded contexts, ensuring log safety and consistency! π
3. Why Developers Prefer spdlog: Top Ten Reasons to Choose It π
- Speed and Performance Advantage: Logging speed and processing efficiency of spdlog significantly outstrip many other libraries, making logging no longer a performance bottleneck! β‘
- Convenient Installation and Integration Options: It provides multiple installation methods, including header-only and CMake build versions, making it ideal for rapidly evolving development environments! π§
- Rich Documentation and Community Support: The documentation is comprehensive, and an active developer community provides great support. Got a problem? The community is always there to help! π
- Multi-Platform Compatibility: spdlog runs on various operating systems such as Linux, Windows, macOS, and Android, ensuring wide applicability. Cross-platform development becomes effortless! π
- Free and Open Source, MIT License: Users can freely use, modify, and distribute the library, offering developers flexibility without worries about copyright issues. The power of open source propels our progress! π
By utilizing spdlog, developers can easily establish an efficient logging system that meets performance and functional demands, making log management simpler and allowing more focus on innovation and optimization of applications.
4. Installing spdlog: Starting from Scratch π
Ready to start using spdlog
? First, you need to set it up in your development environment! You can install spdlog
in various ways, such as cloning the repository from GitHub or integrating it directly with CMake. Letβs take a look at a simple example of how to set up spdlog
with CMake:
CMake Configuration
Create a CMakeLists.txt
file:
cmake_minimum_required(VERSION 3.11)
project(spdlog_examples CXX)
if(NOT TARGET spdlog) # Check if spdlog is installed
find_package(spdlog REQUIRED) # Find and link spdlog library
endif()
# ---------------------------------------------------------------------------------------
# Example using precompiled library
# ---------------------------------------------------------------------------------------
add_executable(example example.cpp) // Create an executable file
target_link_libraries(example PRIVATE spdlog::spdlog $<$<BOOL:${MINGW}>:ws2_32>) // Link spdlog to executable
# ---------------------------------------------------------------------------------------
# Example using header-only library
# ---------------------------------------------------------------------------------------
if(SPDLOG_BUILD_EXAMPLE_HO)
add_executable(example_header_only example.cpp)
target_link_libraries(example_header_only PRIVATE spdlog::spdlog_header_only) // Create an executable for header-only version
endif()
With this configuration file, if you want to build and run an application that uses spdlog
, CMakeLists.txt
will automatically handle linking the library. Super easyβinstalling and managing dependencies becomes a breeze! π
5. Basic Usage of spdlog: Getting Started Easily π
Letβs see the basic usage of spdlog
. The following code example demonstrates different logging levels:
#include "spdlog/spdlog.h" // Include spdlog library
int main()
{
spdlog::info("Welcome to spdlog!"); // Log an informational message
spdlog::error("Some error message with arg: {}", 1); // Log an error message with a parameter
spdlog::warn("Easy padding in numbers like {:08d}", 12); // Log a warning, number padded with zeros
spdlog::critical("Support for int: {0:d}; hex: {0:x}; oct: {0:o}; bin: {0:b}", 42); // Log a critical message with multiple numeral bases
spdlog::info("Support for floats {:03.2f}", 1.23456); // Format and log a floating point number
spdlog::info("Positional args are {1} {0}..", "too", "supported"); // Use positional arguments
spdlog::info("{:<30}", "left aligned"); // Log left-aligned string
spdlog::set_level(spdlog::level::debug); // Set global log level to debug
spdlog::debug("This message should be displayed.."); // Log a debug message
// Change log pattern; customize log output format.
spdlog::set_pattern("[%H:%M:%S %z] [%n] [%^---%L---%$] [thread %t] %v");
// Compile-time log level
SPDLOG_TRACE("Some trace message with param {}", 42); // Trace level message, may not display due to log level settings
SPDLOG_DEBUG("Some debug message"); // Another debug message
}
Code Explanation
In this code, weβve included the spdlog
library. Using different logging methods such as info
, error
, warn
, critical
, and debug
, we log messages at the corresponding levels. Each logging method can be combined with formatting parameters, helping to make the output more intuitive, especially useful for debugging applications!
6. Advanced Usage: Various Logging Methods π
The following example demonstrates how to utilize various logging methods, such as console logging, file logging, rotating logs, and even asynchronous logging.
Example: Console Logging
#include "spdlog/sinks/stdout_color_sinks.h" // Include color console support
void stdout_example()
{
// Create a color console logger with multi-thread support
auto console = spdlog::stdout_color_mt("console");
auto err_logger = spdlog::stderr_color_mt("stderr");
spdlog::get("console")->info("loggers can be retrieved from a global registry using the spdlog::get(logger_name)");
}
Explanation:
With spdlog::stdout_color_mt
, we create a color console logger that supports multi-threading (meaning multiple threads can log to it simultaneously). Using spdlog::get("console")
, we can get the console logger instance by name, making it easy to log messages.
Example: Basic File Logger
#include "spdlog/sinks/basic_file_sink.h" // Include basic file output support
void basic_logfile_example()
{
try
{
auto logger = spdlog::basic_logger_mt("basic_logger", "logs/basic-log.txt"); // Create a logger that logs to a file
}
catch (const spdlog::spdlog_ex &ex) // Catch possible exceptions
{
std::cout << "Log init failed: " << ex.what() << std::endl; // Print error message
}
}
Explanation:
In this code, we create a logger that outputs log messages to a specified file. Handling exceptions ensures that we are notified in case of initialization failure (e.g., due to permission issues).
Example: Rotating Logs
#include "spdlog/sinks/rotating_file_sink.h" // Include rotating file output support
void rotating_example()
{
// Create a rotating file logger, with a maximum size of 5 MB and keep 3 rotating files
auto max_size = 1048576 * 5; // 5 MB
auto max_files = 3; // Keep 3 rotating files.
auto logger = spdlog::rotating_logger_mt("some_logger_name", "logs/rotating.txt", max_size, max_files);
}
Explanation:
The rotating logger automatically creates new log files when the current log file reaches the specified size, enabling you to control log file sizes while retaining old logs.
Example: Daily Logs
#include "spdlog/sinks/daily_file_sink.h" // Include daily file output support
void daily_example()
{
// Create a daily logger - a new file is created every day at 2:30 am
auto logger = spdlog::daily_logger_mt("daily_logger", "logs/daily.txt", 2, 30);
}
Explanation:
The daily logger creates new log files at a specified time every day, which is very useful for applications that require daily archiving!
Example: Asynchronous Logging
#include "spdlog/async.h" // Include asynchronous support
#include "spdlog/sinks/basic_file_sink.h"
void async_example()
{
auto async_file = spdlog::basic_logger_mt<spdlog::async_factory>("async_file_logger", "logs/async_log.txt");
}
Explanation:
Using spdlog::async_factory
creates a logger that can handle log messages in a separate thread, ensuring that logging does not block the main application thread.
7. Advanced Features: Flushing and Backtrace ποΈ
Want to ensure your logs are always saved in a timely manner? You can configure spdlog
to periodically flush logs and backtrack debugging messages:
// Flush all registered loggers every 3 seconds
spdlog::flush_every(std::chrono::seconds(3));
Explanation:
This code ensures that all buffered logs are written every three seconds before the program crashes, minimizing log loss.
// Debug messages can be stored in a circular buffer instead of being logged right away
spdlog::enable_backtrace(32); // Store the latest 32 messages
for(int i = 0; i < 100; i++)
{
spdlog::debug("Backtrace message {}", i); // Not logged yet..
}
// For example, if an error occurs:
spdlog::dump_backtrace(); // Now log them! Show the last 32 messages
Explanation:
The above code shows how to capture backup debugging messages for later logging. This is very useful for debugging, allowing detailed debugging information to be logged when needed.
8. Logging User-Defined Types π οΈ
Want to log messages from custom types? You can absolutely do so! Hereβs an example:
template<>
struct fmt::formatter<my_type> : fmt::formatter<std::string>
{
auto format(my_type my, format_context &ctx) const -> decltype(ctx.out())
{
return fmt::format_to(ctx.out(), "[my_type i={}]", my.i); // Custom formatting output
}
};
void user_defined_example()
{
spdlog::info("user defined type: {}", my_type(14)); // Log custom type
}
Explanation:
In this example, we specialize the formatter template to define how to log a custom type my_type
. This makes integration with spdlog
for custom types smooth and seamless.
This article provides detailed steps and code examples to help you effortlessly get started with the powerful spdlog
C++ logging library. I hope these examples and explanations assist you in utilizing spdlog
effectively, delivering robust logging capabilities for your applications! π